Here is an EXE in case you trust me not to destroy your computer: Project1.exe
Code
#include <iostream>
using namespace std;
int field[3][3];
string input;
int coordX, coordY;
bool gameOn = 1;
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
int turn(int player)
{
bool turn = 1;
do
{
do
{
coordX = inputINT("X coordinate: ");
if (!(coordX >=0 && coordX <= 2))
cout << "ERROR: Out of range." << endl;
} while (!(coordX >=0 && coordX <= 2));
do
{
coordY = inputINT("Y coordinate: ");
if (!(coordY >=0 && coordY <= 2))
cout << "ERROR: Out of range." << endl;
} while (!(coordY >=0 && coordY <= 2));
if (field[coordX][coordY] == 0)
{
field[coordX][coordY] = player;
turn = 0;
}
else
cout << "ERROR: Cannot place marker there." << endl;
} while (turn);
}
int result()
{
return 0;
}
void clrScrn()
{
for (int i = 0; i < 50; i++)
{
cout << endl;
}
}
void printField()
{
cout << " 0 1 2" << endl;
for (int x = 0; x < 3; x++)
{
cout << x << " ";
for (int y = 0; y < 3; y++)
{
switch (field[y][x])
{
case 0:
cout << "- ";
break;
case 1:
cout << "X ";
break;
case 10:
cout << "O ";
break;
}
}
cout << endl;
}
}
int winChk(int sum)
{
if (sum == 3)
{
cout << "Player 1 wins!" << endl;
return 1; // p1 win
}
if (sum == 30)
{
cout << "Player 2 wins!" << endl;
return 10; // p2 win
}
return 0;
}
int gameState()
{
int sum, r;
for (int x = 0; x < 3; x++) //check rows
{
sum = 0;
for (int y = 0; y < 3; y++)
{
sum += field[x][y];
r = winChk(sum);
if (r != 0)
return r;
}
}
for (int x = 0; x < 3; x++) // check columns
{
sum = 0;
for (int y = 0; y < 3; y++)
{
sum += field[y][x];
r = winChk(sum);
if (r != 0)
return r;
}
}
sum = 0; //check diagonal 1
for (int x = 0; x < 3; x++)
sum += field[x][x];
r = winChk(sum);
if (r != 0)
return r;
sum = 0; //check diagonal 2
for (int x = 2; x >= 0; x--)
sum += field[x][2-x];
r = winChk(sum);
if (r != 0)
return r;
return 0; // continue
}
int main()
{
while (gameOn)
{
if (gameState() == 0)
{
clrScrn();
printField();
cout << endl;
cout << "Player 1" << endl;
turn(1);
}
else
gameOn = 0;
clrScrn();
printField();
if (gameState() == 0)
{
cout << endl;
cout << "Player 2" << endl;
turn(10);
}
else
gameOn = 0;
}
cout << endl;
system("PAUSE");
return EXIT_SUCCESS;
}
using namespace std;
int field[3][3];
string input;
int coordX, coordY;
bool gameOn = 1;
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
int turn(int player)
{
bool turn = 1;
do
{
do
{
coordX = inputINT("X coordinate: ");
if (!(coordX >=0 && coordX <= 2))
cout << "ERROR: Out of range." << endl;
} while (!(coordX >=0 && coordX <= 2));
do
{
coordY = inputINT("Y coordinate: ");
if (!(coordY >=0 && coordY <= 2))
cout << "ERROR: Out of range." << endl;
} while (!(coordY >=0 && coordY <= 2));
if (field[coordX][coordY] == 0)
{
field[coordX][coordY] = player;
turn = 0;
}
else
cout << "ERROR: Cannot place marker there." << endl;
} while (turn);
}
int result()
{
return 0;
}
void clrScrn()
{
for (int i = 0; i < 50; i++)
{
cout << endl;
}
}
void printField()
{
cout << " 0 1 2" << endl;
for (int x = 0; x < 3; x++)
{
cout << x << " ";
for (int y = 0; y < 3; y++)
{
switch (field[y][x])
{
case 0:
cout << "- ";
break;
case 1:
cout << "X ";
break;
case 10:
cout << "O ";
break;
}
}
cout << endl;
}
}
int winChk(int sum)
{
if (sum == 3)
{
cout << "Player 1 wins!" << endl;
return 1; // p1 win
}
if (sum == 30)
{
cout << "Player 2 wins!" << endl;
return 10; // p2 win
}
return 0;
}
int gameState()
{
int sum, r;
for (int x = 0; x < 3; x++) //check rows
{
sum = 0;
for (int y = 0; y < 3; y++)
{
sum += field[x][y];
r = winChk(sum);
if (r != 0)
return r;
}
}
for (int x = 0; x < 3; x++) // check columns
{
sum = 0;
for (int y = 0; y < 3; y++)
{
sum += field[y][x];
r = winChk(sum);
if (r != 0)
return r;
}
}
sum = 0; //check diagonal 1
for (int x = 0; x < 3; x++)
sum += field[x][x];
r = winChk(sum);
if (r != 0)
return r;
sum = 0; //check diagonal 2
for (int x = 2; x >= 0; x--)
sum += field[x][2-x];
r = winChk(sum);
if (r != 0)
return r;
return 0; // continue
}
int main()
{
while (gameOn)
{
if (gameState() == 0)
{
clrScrn();
printField();
cout << endl;
cout << "Player 1" << endl;
turn(1);
}
else
gameOn = 0;
clrScrn();
printField();
if (gameState() == 0)
{
cout << endl;
cout << "Player 2" << endl;
turn(10);
}
else
gameOn = 0;
}
cout << endl;
system("PAUSE");
return EXIT_SUCCESS;
}
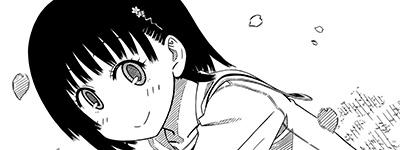