What I'm trying to do is create a vector of CRegularPolygons. Then, using another function, add objects of CRegularPolygons to the vector. Then, in the highlighted line of code, I'm trying to access a function of an object of the vector.
What am I doing wrong? (I suspect it has something to do with how I have attempted to utilize pointers.)
Code
#include <cstdlib>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class CRegularPolygon
{
string name;
int numSides;
float lenSide, apothem;
public:
CRegularPolygon(string name_, int numSides_, float lenSide_, float apothem_)
{
name = name_;
numSides = numSides_;
lenSide = lenSide_;
apothem = apothem_;
}
float perimeter()
{
return numSides * lenSide;
}
float area()
{
return 0.5 * numSides * lenSide * apothem;
}
float internalAngle()
{
return (numSides - 2) / numSides * 180;
}
int internalAngleSum()
{
return (numSides - 2) * 180;
}
};
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
float inputFLOAT(string prompt)
{
float n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
void createRegularPolygons(vector<CRegularPolygon> regPolys)
{
string name;
int numSides;
float lenSide, apothem;
string createRegPoly;
do
{
cout << "Create a new regular polygon? (y/n)" << endl;
cin >> createRegPoly;
if(createRegPoly == "y")
{
// gather statistics of polygon
cout << "Enter ID/name of polygon:" << endl;
cin >> name;
numSides = inputINT("Enter number of sides:\n");
lenSide = inputFLOAT("Enter length of one side:\n");
apothem = inputFLOAT("Enter apothem\n(the line segment from the center of the regular polygon to the\nmidpoint of a side):\n");
regPolys.push_back(CRegularPolygon(name, numSides, lenSide, apothem)); // create polygon
}
} while(createRegPoly == "y");
}
int main()
{
vector<CRegularPolygon> regularPolygons;
vector<CRegularPolygon> *regPolys = ®ularPolygons;
createRegularPolygons(*regPolys);
cout << (regularPolygons.at(0).internalAngle()) << endl; // PROBLEMATIC LINE
system("PAUSE");
return 0;
}
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class CRegularPolygon
{
string name;
int numSides;
float lenSide, apothem;
public:
CRegularPolygon(string name_, int numSides_, float lenSide_, float apothem_)
{
name = name_;
numSides = numSides_;
lenSide = lenSide_;
apothem = apothem_;
}
float perimeter()
{
return numSides * lenSide;
}
float area()
{
return 0.5 * numSides * lenSide * apothem;
}
float internalAngle()
{
return (numSides - 2) / numSides * 180;
}
int internalAngleSum()
{
return (numSides - 2) * 180;
}
};
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
float inputFLOAT(string prompt)
{
float n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
void createRegularPolygons(vector<CRegularPolygon> regPolys)
{
string name;
int numSides;
float lenSide, apothem;
string createRegPoly;
do
{
cout << "Create a new regular polygon? (y/n)" << endl;
cin >> createRegPoly;
if(createRegPoly == "y")
{
// gather statistics of polygon
cout << "Enter ID/name of polygon:" << endl;
cin >> name;
numSides = inputINT("Enter number of sides:\n");
lenSide = inputFLOAT("Enter length of one side:\n");
apothem = inputFLOAT("Enter apothem\n(the line segment from the center of the regular polygon to the\nmidpoint of a side):\n");
regPolys.push_back(CRegularPolygon(name, numSides, lenSide, apothem)); // create polygon
}
} while(createRegPoly == "y");
}
int main()
{
vector<CRegularPolygon> regularPolygons;
vector<CRegularPolygon> *regPolys = ®ularPolygons;
createRegularPolygons(*regPolys);
cout << (regularPolygons.at(0).internalAngle()) << endl; // PROBLEMATIC LINE
system("PAUSE");
return 0;
}
EDIT:
nvm I fixed my pointer work I think
Code
#include <cstdlib>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class CRegularPolygon
{
string name;
int numSides;
float lenSide, apothem;
public:
CRegularPolygon(string name_, int numSides_, float lenSide_, float apothem_)
{
name = name_;
numSides = numSides_;
lenSide = lenSide_;
apothem = apothem_;
}
float perimeter()
{
return numSides * lenSide;
}
float area()
{
return 0.5 * numSides * lenSide * apothem;
}
float internalAngle()
{
return (numSides - 2) / numSides * 180;
}
int internalAngleSum()
{
return (numSides - 2) * 180;
}
};
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
float inputFLOAT(string prompt)
{
float n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
void createRegularPolygons(vector<CRegularPolygon> *regPolys)
{
string name;
int numSides;
float lenSide, apothem;
string createRegPoly;
do
{
cout << "Create a new regular polygon? (y/n)" << endl;
cin >> createRegPoly;
if(createRegPoly == "y")
{
// gather statistics of polygon
cout << "Enter ID/name of polygon:" << endl;
cin >> name;
numSides = inputINT("Enter number of sides:\n");
lenSide = inputFLOAT("Enter length of one side:\n");
apothem = inputFLOAT("Enter apothem\n(the line segment from the center of the regular polygon to the\nmidpoint of a side):\n");
regPolys->push_back(CRegularPolygon(name, numSides, lenSide, apothem)); // create polygon
}
} while(createRegPoly == "y");
}
int main()
{
vector<CRegularPolygon> regularPolygons;
vector<CRegularPolygon> *regPolys = ®ularPolygons;
createRegularPolygons(regPolys);
cout << regularPolygons.at(0).internalAngle() << endl;
system("PAUSE");
return 0;
}
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class CRegularPolygon
{
string name;
int numSides;
float lenSide, apothem;
public:
CRegularPolygon(string name_, int numSides_, float lenSide_, float apothem_)
{
name = name_;
numSides = numSides_;
lenSide = lenSide_;
apothem = apothem_;
}
float perimeter()
{
return numSides * lenSide;
}
float area()
{
return 0.5 * numSides * lenSide * apothem;
}
float internalAngle()
{
return (numSides - 2) / numSides * 180;
}
int internalAngleSum()
{
return (numSides - 2) * 180;
}
};
int inputINT(string prompt)
{
int n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
float inputFLOAT(string prompt)
{
float n;
bool bad = 1;
do
{
cout << prompt;
cin >> n;
if (cin.fail())
{
cout << "ERROR: Invalid input." << endl;
cin.clear();
cin.ignore(numeric_limits<int>::max(),'\n');
}
else
bad = 0;
} while (bad);
return n;
}
void createRegularPolygons(vector<CRegularPolygon> *regPolys)
{
string name;
int numSides;
float lenSide, apothem;
string createRegPoly;
do
{
cout << "Create a new regular polygon? (y/n)" << endl;
cin >> createRegPoly;
if(createRegPoly == "y")
{
// gather statistics of polygon
cout << "Enter ID/name of polygon:" << endl;
cin >> name;
numSides = inputINT("Enter number of sides:\n");
lenSide = inputFLOAT("Enter length of one side:\n");
apothem = inputFLOAT("Enter apothem\n(the line segment from the center of the regular polygon to the\nmidpoint of a side):\n");
regPolys->push_back(CRegularPolygon(name, numSides, lenSide, apothem)); // create polygon
}
} while(createRegPoly == "y");
}
int main()
{
vector<CRegularPolygon> regularPolygons;
vector<CRegularPolygon> *regPolys = ®ularPolygons;
createRegularPolygons(regPolys);
cout << regularPolygons.at(0).internalAngle() << endl;
system("PAUSE");
return 0;
}
Could someone explain to me what I was doing wrong at first?
EDIT2:
Also, are there commands/functions that returns the maximum values of int, float, double, etc.?
Post has been edited 2 time(s), last time on Dec 10 2011, 2:34 am by Sand Wraith.
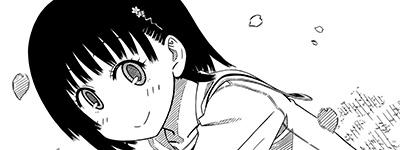