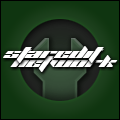
Relatively ancient and inactive
Well, basically, we're supposed to create custom number systems in Scheme. Which I think is completely pointless, but no one cares, so.. Well, anyway, I chose to do a number system with positive/negative integer complex numbers. Basically, we're supposed to create addition, subtraction, quotient and multiplication functions, as well as one of the more interesting functions like sqrt or exp. If someone could help me troubleshoot this, it'd be great. Scheme's not helping at all.
(define add ;(# #)
(lambda (a b)
(cond
((and (equal? (car a) 0) (equal? (car (cdr a)) 0)) b)
((and (equal? (car b) 0) (equal? (car (cdr b)) 0)) a)
(else (add ((cond ((> (car b) 0) (+ 1 (car a)))
((equal? (car b) 0) (car a))
(else (- (car a) 1)))
(cond ((> (car (cdr b)) 0) (+ (car (cdr a)) 1))
((equal? (car (cdr b)) 0) (car (cdr a)))
(else (- (car (cdr a)) 1))))
((cond ((> (car b) 0)(- (car b) 1))
((equal? (car b) 0) (car b))
(else (+ (car b) 1)))
(cond ((> (car (cdr b)) 0)(- (car (cdr b)) 1))
((equal? (car (cdr b)) 0)(car (cdr b)))
(else (+ (car (cdr b)) 1)))))))))
'
This is the adding function (from which I can probably derive everything else, or at least learn from it). It doesn't work. The complex numbers are supposed to go like this: (# #). The first one is a positive/negative real number, the second is positive/negative imaginary number. What I try to do here is basically create a recursive loop that keeps either increasing/decreasing the b list to (0 0) While increasing/decreasing the A list accordingly.
Any help much appreciated

.
None.
I can give you a pointer on how NOT to do your calculator:
Calculators gone badCan you do bitwise operations in Scheme? That could help (although I'm not sure if the way I'm thinking it could be implemented isn't a huge wtf by itself) Or bitshift, although I don't quite see how to implement that off top of my head.
None.
You've probably misplaced a parenthesis

Expansion:
(define add ;(# #)
(lambda (a b)
(cond
((and (equal? (car a) 0) (equal? (car (cdr a)) 0)) b)
((and (equal? (car b) 0) (equal? (car (cdr b)) 0)) a)
(else
(add
(
(cond ((> (car b) 0) (+ 1 (car a)))
((equal? (car b) 0) (car a))
(else (- (car a) 1)))
(cond ((> (car (cdr b)) 0) (+ (car (cdr a)) 1))
((equal? (car (cdr b)) 0) (car (cdr a)))
(else (- (car (cdr a)) 1)))
)
(
(cond ((> (car b) 0)(- (car b) 1))
((equal? (car b) 0) (car b))
(else (+ (car b) 1)))
(cond ((> (car (cdr b)) 0)(- (car (cdr b)) 1))
((equal? (car (cdr b)) 0)(car (cdr b)))
(else (+ (car (cdr b)) 1)))
)
)
)
)
)
)
Post has been edited 5 time(s), last time on Mar 29 2008, 7:51 pm by Doodle77.
None.
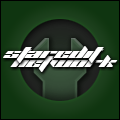
Relatively ancient and inactive
Produces errors.
(add (3 4) (3 4))
{bug} procedure application: expected procedure, given: 3; arguments were: 4
With the most unhelpful error message ever.
Can you do bitwise operations in Scheme? That could help (although I'm not sure if the way I'm thinking it could be implemented isn't a huge wtf by itself) Or bitshift, although I don't quite see how to implement that off top of my head.
Scheme's a bit unorthadox a language. We can't use bitwise operations or anything like that - just basics of what you learn in two months of Scheme in a classroom of 30 students.
Post has been edited 1 time(s), last time on Mar 29 2008, 9:18 pm by Centreri.
None.
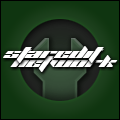
Relatively ancient and inactive
Jesus, navigating through those mounds of parenthases and succeeding must make me a genius. My successful answer:
(define add ;(# #)
(lambda (a b)
(cond
((and (equal? (car a) 0) (equal? (car (cdr a)) 0)) b)
((and (equal? (car b) 0) (equal? (car (cdr b)) 0)) a)
(else (add (cons (cond ((> (car b) 0) (+ 1 (car a)))
((equal? (car b) 0) (car a))
(else (- (car a) 1)))
(cons (cond ((> (car (cdr b)) 0) (+ (car (cdr a)) 1))
((equal? (car (cdr b)) 0) (car (cdr a)))
(else (- (car (cdr a)) 1))) '()))
(cons (cond ((> (car b) 0)(- (car b) 1))
((equal? (car b) 0) (car b))
(else (+ (car b) 1)))
(cons (cond ((> (car (cdr b)) 0)(- (car (cdr b)) 1))
((equal? (car (cdr b)) 0)(car (cdr b)))
(else (+ (car (cdr b)) 1))) '())))))))
This thread is now dedicated to asking Scheme-related questions and praising my awesomeness. Mostly praising my awesomeness. Let the 1337/\/355 commence.
Updates: Things started moving really quickly with add out of the way. Here come Subtract and Multiply!
(define subtract
(lambda (a b)
(add a (cons (- 0 (car b)) (cons (- 0 (car (cdr b))) '())))))
(define multiply
(lambda (a b)
(cons (+ (* (car a) (car b)) (* -1 (car (cdr a)) (car (cdr b))))
(cons (+ (* (car a) (car (cdr b))) (* (car (cdr a)) (car b))) '()))))
EDIT: I feel 100% retarded. I went through all the work making the Add function, when this would suffice...
(define add2 ;I felt so stupid making the first function after and realizing I could've done it like this...
(lambda (a b)
(cons (+ (car a) (car b)) (cons (+ (car (cdr a)) (car (cdr b))) '()))))
Post has been edited 2 time(s), last time on Mar 29 2008, 10:11 pm by Centreri.
None.
Gah all those parenthesis (is the plural the same as the singular?) hurt my eyes. Why do you even have to learn Scheme in class? Heck, implementing a math system looks easier in SC triggers than in Scheme.
None.
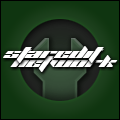
Relatively ancient and inactive
I'm very sad. Check my 2nd edit. I spent so much time on that Add function, when it was completely obsolete. Well, I might get a few extra points for it

.
None.
You should have worsened it for the next OMGWTF contest over at thedailywtf.com, or for the corrector's pleasure at looking at a horror which works (leave the simpler version as some "beta" work or something and never call it).
None.
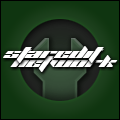
Relatively ancient and inactive
Worsened it? Don't make me do it -.-.
None.